News
Could AI Agents Create a New Crypto Economy?
Jul 4, 2025 • 0 • BitfinexUSDC deposits and withdrawals now available on Sonic!
Jul 4, 2025 • 0 • KrakenChart Decoder Series: Stochastic Oscillator – The Trader’s Radar for Reversals
Jul 4, 2025 • 0 • BitfinexKraken assists U.S. Secret Service in record-breaking crypto fraud seizure
Jul 3, 2025 • 0 • KrakenHTX WEEKLY:6 Jul.202
6 hours ago • 0 • HuobiCould AI Agents Create a New Crypto Economy?
Jul 4, 2025 • 0 • BitfinexUSDC deposits and withdrawals now available on Sonic!
Jul 4, 2025 • 0 • KrakenChart Decoder Series: Stochastic Oscillator – The Trader’s Radar for Reversals
Welcome back to the Chart Decoder Series, your guide to mastering the essential tools for reading Bitfinex charts with precision.
Kraken assists U.S. Secret Service in record-breaking crypto fraud seizure
Jul 3, 2025 • 0 • KrakenICNT is available for trading!
Jul 3, 2025 • 0 • KrakenMERL is available for trading!
Jul 3, 2025 • 0 • KrakenHTX Celebrates 12th Anniversary with Grand Launch of Million-Dollar HTTC S1 Trading Competition
Jul 3, 2025 • 0 • HuobiFirst U.S. Solana Staking ETF Debuts with $33M in Volume
Jul 3, 2025 • 1 view • Coinbase ProMemeCore (M) is available for trading!
Jul 3, 2025 • 0 • KrakenHTX DAO Showcases Compliance and Decentralized Governance Vision at Istanbul Blockchain Week
Jul 3, 2025 • 0 • HuobiHow to Set Up and Use Trust Wallet for Binance Smart Chain
Oct 30, 2020 • 188,008 views • BinanceYour Essential Guide To Binance Leveraged Tokens
Aug 13, 2020 • 126,090 views • BinanceHow to Sell Your Bitcoin Into Cash on Binance (2021 Update)
Feb 8, 2021 • 111,635 views • BinanceWhat is Grid Trading? (A Crypto-Futures Guide)
Grid trading is a robot that automates the buying and selling of futures contracts.
Kraken Introduces No-Fee USD Deposits with Signature and MVB Bank
Aug 5, 2020 • 66,430 views • KrakenBacktest Your Trading Strategy With Binance Futures Historical Data
Oct 23, 2020 • 65,085 views • BinanceHow to Buy Bitcoin with South Africa’s FNB E-wallet on Binance P2P
Oct 27, 2020 • 51,292 views • BinanceWeb 3.0 and How To Invest In It Through Crypto
Jul 30, 2021 • 41,879 views • BinanceBecome a VIP Trader on Binance and Enjoy Unique Benefits
Nov 18, 2020 • 38,587 views • Binance3 Tips To Trade Leveraged Tokens in Trending Markets
Nov 13, 2020 • 37,270 views • BinanceA Beginner’s Guide To Funding Rates
Oct 14, 2020 • 29,688 views • BinanceNews of the Week
Kraken rising: #2 in Kaiko’s Q2 2025 Exchange Ranking
Jul 1, 2025 • 5 min read- #Bitcoin#Staking#Bitcoins Spot ETF+2 more tags
First U.S. Solana Staking ETF Debuts with $33M in Volume
Jul 3, 2025 • 2 min read HTX WEEKLY:29 Jun. 2025
Jun 30, 2025 • 1 min readReimagining Financial Infrastructure: How Stablecoins Are Quietly Reshaping Global Value Flows
Jun 30, 2025 • 5 min read
Start trading with Cryptohopper for free!
Free to use - no credit card required
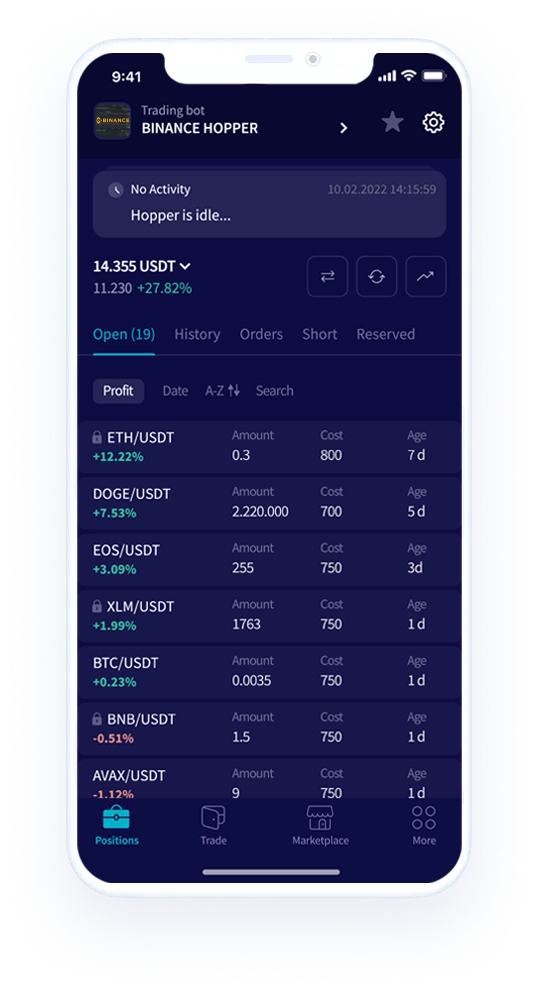
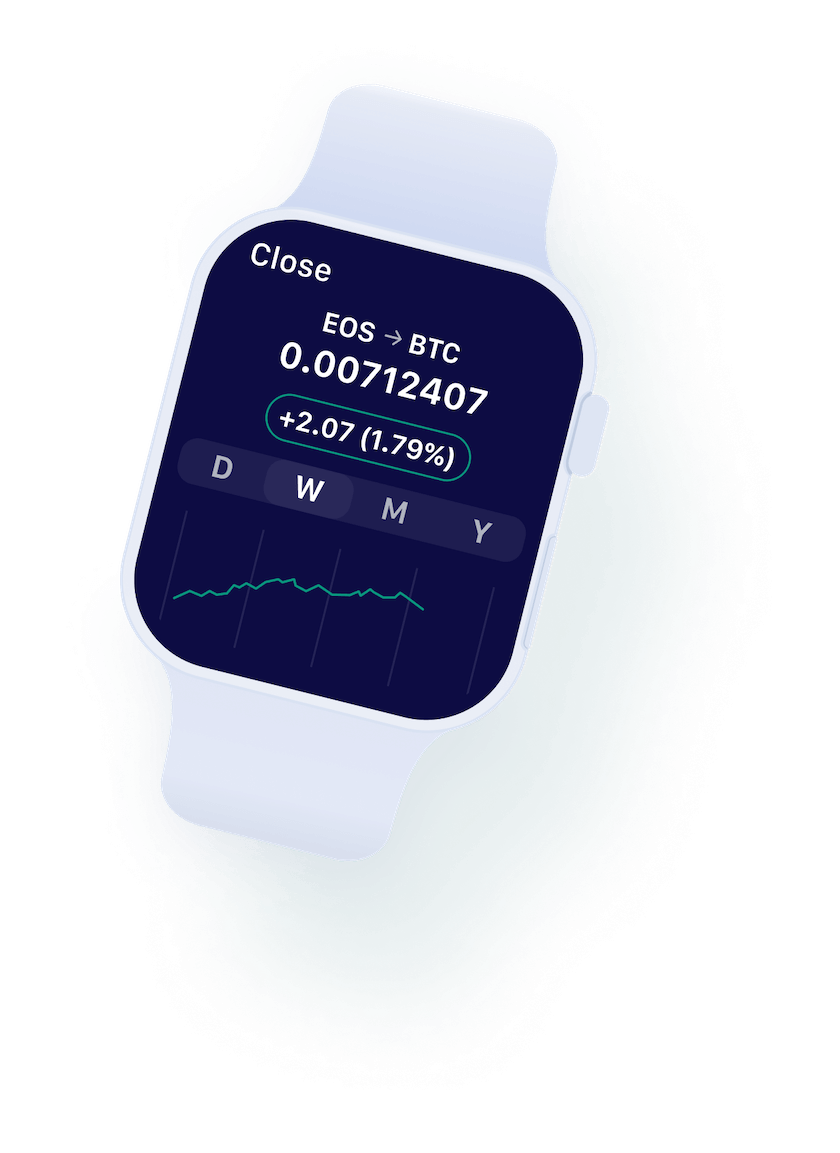